Listen up, developers and tech enthusiasts! If you're diving into the world of web development, there's one name you absolutely need to know: Express.js. Yeah, you heard that right. Express is not just another framework; it's a game-changer for building web applications with Node.js. So, buckle up because we're about to break it down for you in a way that'll make your coding journey smoother and more exciting.
In today's fast-paced digital world, having a solid foundation for your web apps is crucial. That's where Express.js comes in. It's like the Swiss Army knife of web frameworks—compact, versatile, and ready to handle whatever you throw at it. Whether you're a beginner just starting out or a seasoned pro looking for something reliable, Express has got your back.
Now, before we dive deep into the nitty-gritty, let's set the stage. Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. But don't worry, we'll unpack all of that later. For now, just know that it's powerful, easy to use, and trusted by developers worldwide. Ready to learn more? Let's get started!
Read also:Ski Bri Onlyfans Leaks The Untold Story You Need To Hear
What Exactly Is Express.js?
Alright, let's get down to business. Express.js, or simply Express, is a lightweight Node.js framework that simplifies the process of building web applications. Think of it as the backbone of your app, providing the structure and tools you need to create dynamic, scalable, and efficient websites.
Here's the kicker: Express doesn't force you into a rigid structure. Instead, it gives you the freedom to design your app the way you want. It's like having a blank canvas where you can paint your masterpiece. And trust me, that flexibility is a game-changer when it comes to creativity and innovation.
Some key features that make Express stand out include routing, middleware support, template engines, and a whole lot more. We'll explore these features in detail as we move forward, but for now, just know that Express is built to adapt to your needs, not the other way around.
Why Should You Choose Express.js?
Let's talk about why Express.js is the go-to choice for so many developers. First off, it's lightweight. No unnecessary bloat here. Just the essentials you need to get the job done. This means faster load times and better performance for your apps.
Then there's the flexibility factor. Unlike some frameworks that try to hold your hand through every step, Express lets you take the reins. You can customize it to fit your specific requirements, which is a huge plus when you're working on complex projects.
But wait, there's more! Express has an active and supportive community. Need help? Chances are someone else has already faced the same challenge and can offer a solution. Plus, with its compatibility with various middleware and third-party libraries, the possibilities are virtually endless.
Read also:Jc Wilds Marilyn Johnson The Untold Story Of Two Icons
Express.js: The Developer's Best Friend
Express isn't just a framework; it's a developer's best friend. It streamlines the development process, making it faster and more efficient. Whether you're building a simple REST API or a complex web app, Express has the tools you need to succeed.
One of the coolest things about Express is its middleware system. Middleware functions are like the glue that holds your app together. They allow you to add functionality, handle requests and responses, and even modify the behavior of your app. It's like having a toolbox full of handy gadgets that you can use whenever you need them.
And let's not forget about scalability. Express is built to grow with your app. As your user base expands and your app becomes more complex, Express can handle the load without breaking a sweat. This makes it an excellent choice for both startups and established companies.
Setting Up Express.js: A Step-by-Step Guide
Now that you know why Express.js is such a big deal, let's talk about how to set it up. Don't worry; it's not as complicated as it might sound. In fact, with a few simple steps, you'll have your Express app up and running in no time.
First things first, you'll need to have Node.js installed on your machine. If you don't have it yet, head over to the official Node.js website and grab the latest version. Once you've got Node.js installed, open up your terminal or command prompt and run the following command:
npm install express
That's it! You've just installed Express.js. Now, let's create a simple app to see it in action. Create a new file called app.js
and add the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => res.send('Hello World!'));
app.listen(port, () => console.log(`Example app listening on port ${port}!`));
Save the file and run it using the command node app.js
. Open your browser and navigate to http://localhost:3000
, and you should see the message "Hello World!" displayed on the screen. Congratulations, you've just created your first Express app!
Key Features of Express.js
Express.js comes packed with features that make it a top choice for developers. Let's take a closer look at some of these features:
- Routing: Express provides a powerful routing system that allows you to define different endpoints for your app. This makes it easy to organize your code and handle different types of requests.
- Middlewares: As we mentioned earlier, middleware functions are a crucial part of Express. They allow you to add functionality to your app, handle errors, and modify requests and responses.
- Template Engines: Express supports various template engines, such as EJS, Pug, and Handlebars. These engines make it easier to generate dynamic HTML pages for your app.
- Scalability: Express is designed to handle large-scale applications. Its modular architecture allows you to add or remove components as needed, ensuring that your app remains efficient and performant.
Understanding Middleware in Express.js
Middlewares are like the unsung heroes of Express.js. They work behind the scenes to make your app function smoothly. There are different types of middleware, including application-level, router-level, and error-handling middleware.
Application-level middleware is attached to the Express app object and is executed for every request to your app. Router-level middleware, on the other hand, is specific to a particular router instance. And finally, error-handling middleware is used to catch and handle errors that occur during the execution of your app.
Here's a simple example of how to use middleware in Express:
app.use((req, res, next) => {
console.log('Time:', Date.now());
next();
});
In this example, we're using a middleware function to log the current time whenever a request is made to the app. The next()
function is used to pass control to the next middleware function in the stack.
Building REST APIs with Express.js
One of the most common use cases for Express.js is building REST APIs. REST (Representational State Transfer) is a popular architectural style for designing networked applications. With Express, you can create APIs that allow different parts of your app—or even other apps—to communicate with each other.
Here's how you can create a simple REST API using Express:
const express = require('express');
const app = express();
const port = 3000;
let users = [{ id: 1, name: 'John Doe' }, { id: 2, name: 'Jane Doe' }];
app.get('/users', (req, res) => res.send(users));
app.listen(port, () => console.log(`API listening on port ${port}!`));
In this example, we're creating an API endpoint that returns a list of users. You can expand this API by adding more endpoints and functionality as needed.
Best Practices for Building REST APIs with Express.js
When building REST APIs with Express.js, there are a few best practices you should keep in mind:
- Use meaningful endpoint names: Your API endpoints should be self-explanatory and follow a consistent naming convention.
- Handle errors gracefully: Make sure to handle errors properly and return appropriate HTTP status codes.
- Secure your API: Use authentication and authorization mechanisms to protect your API from unauthorized access.
- Optimize performance: Use caching, compression, and other techniques to improve the performance of your API.
Express.js vs Other Frameworks
Now, you might be wondering how Express.js stacks up against other web frameworks. Well, let's take a look:
- Express.js: Lightweight, flexible, and easy to use. Perfect for building both simple and complex web apps.
- Rails: A full-stack framework for Ruby that provides a lot of built-in functionality. Great for rapid development, but can be overkill for smaller projects.
- Django: A high-level Python web framework that emphasizes rapid development and clean design. Similar to Rails, it's ideal for larger projects.
Each framework has its strengths and weaknesses, so the best choice depends on your specific needs and preferences. However, if you're working with Node.js, Express.js is definitely worth considering.
Why Developers Love Express.js
Developers love Express.js for many reasons. It's lightweight, which means it doesn't slow down your app with unnecessary features. It's flexible, allowing you to customize it to fit your needs. And it's easy to learn, making it accessible to developers of all skill levels.
Plus, with its strong community support and compatibility with various tools and libraries, Express is a framework that keeps on giving. Whether you're building a small personal project or a large enterprise application, Express has the tools and resources you need to succeed.
Common Challenges and Solutions
While Express.js is a fantastic framework, it does come with its own set of challenges. Here are some common issues developers face and how to overcome them:
- Performance optimization: As your app grows, you may encounter performance issues. To tackle this, use tools like caching, compression, and load balancing.
- Security vulnerabilities: Protect your app from common security threats by using secure coding practices, authentication, and encryption.
- Debugging: Debugging can be tricky, but using tools like Node.js Debugger and logging libraries can make the process easier.
Tips for Mastering Express.js
If you're looking to master Express.js, here are a few tips to get you started:
- Practice, practice, practice: The more you code with Express, the better you'll become. Build different types of projects to gain experience.
- Stay updated: The web development landscape is constantly evolving. Keep up with the latest trends and updates in the Express ecosystem.
- Join the community: Connect with other developers, ask questions, and share your knowledge. The Express community is full of helpful and supportive people.
Conclusion: Your Journey with Express.js
So there you have it, folks. Express.js is a minimal and flexible Node.js web application framework that offers a world of possibilities for developers. Whether you're building a simple website or a complex web app, Express has the tools and features you need to succeed.
Remember, the key to mastering any framework is practice and perseverance. Don't be afraid to experiment, make mistakes, and learn from them. And most importantly, have fun while you're at it!
Now it's your turn. What are you waiting for? Dive into the world of Express.js and start building your next big project. And don't forget to share your experiences and creations with the community. Who knows? You might just inspire someone else to take the leap into web development!
Table of Contents
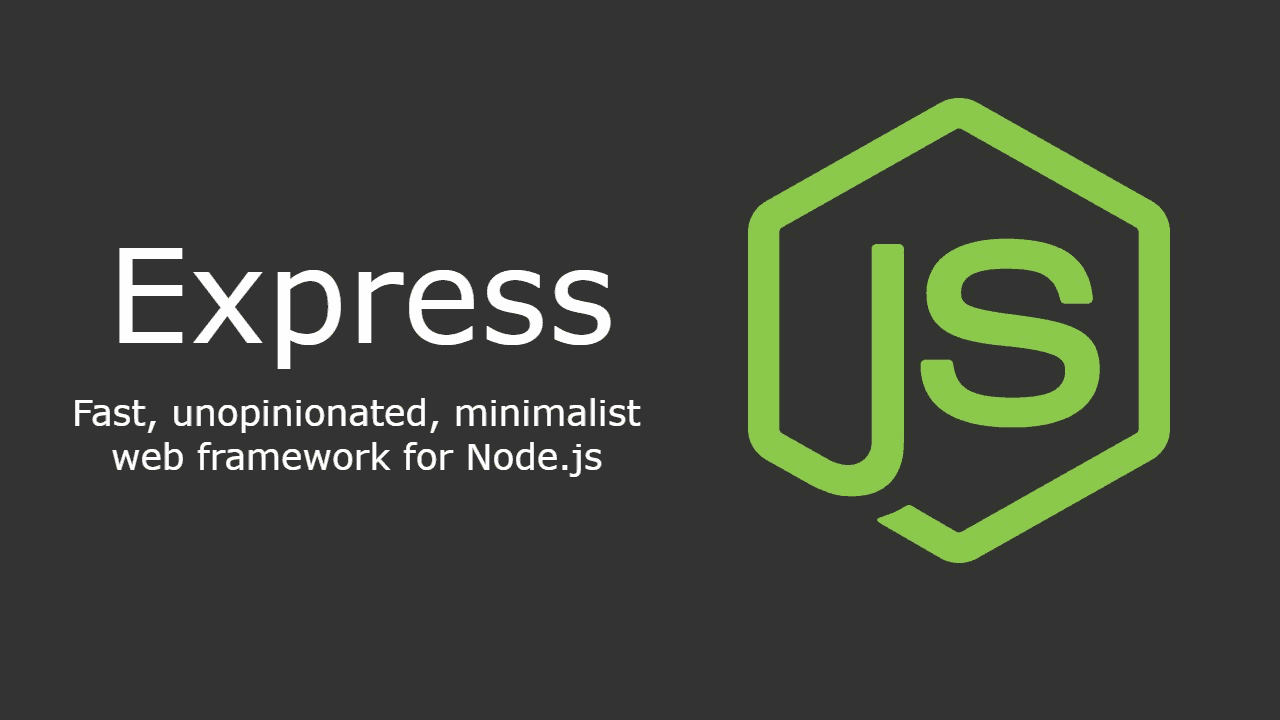

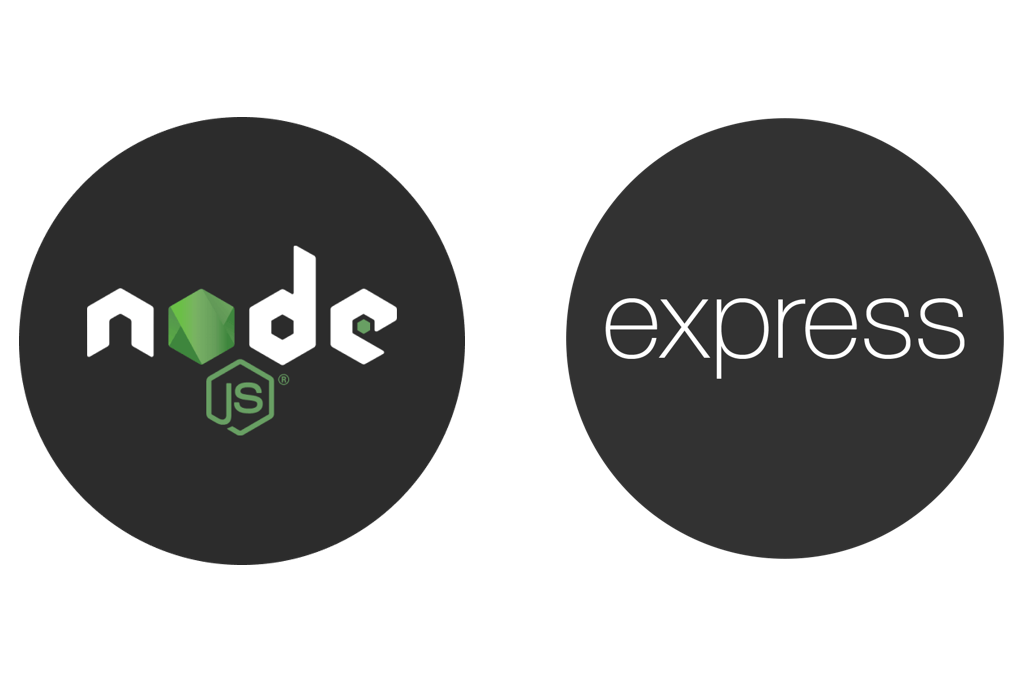